How to publish a post to LinkedIn via API - Part 2: Post a text to LinkedIn API using access token
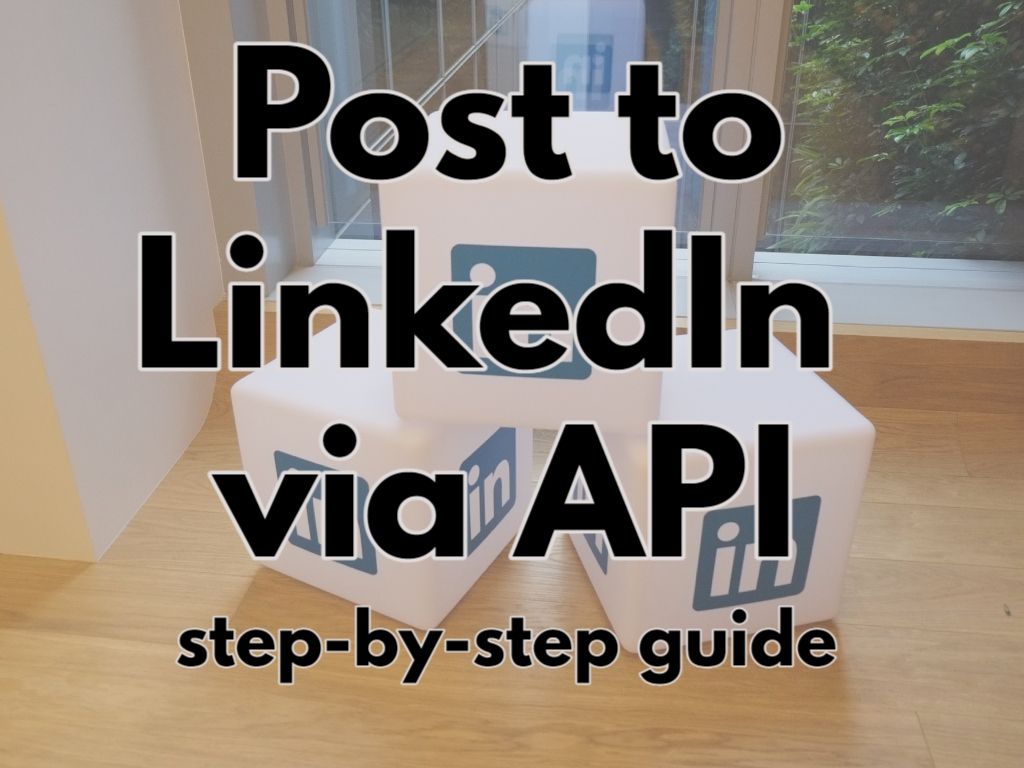
Hi and welcome back π
Introduction
To quickly summarize the previous part, we covered what an API is, what the LinkedIn API is, and how to generate an API access token. The essential requirements before proceeding are an API access token and a LinkedIn user identifier.
This article will show how to use API access token & user identifier to post "This is my first article posted from my Node.js app" to your LinkedIn profile.
Cool? Let's go.
Implementation
To post on LinkedIn via API, all we have to do is call a specific API endpoint with specific parameters. You can use whatever programming language you prefer, but ultimately it boils down to:
1- URL: the URL which is composed of the base API URL and the post path:
https://api.linkedin.com/rest/post
2-Method: the HTTP method we have to use:
POST
3- Headers: the metadata we have to attach to our HTTP request:
{
'LinkedIn-Version': '202210',
'X-Restli-Protocol-Version': '2.0.0',
'Authorization': ' Bearer ___access_token___'
}
4-Body: The content of our post should be formatted as per LinkedIn's specifications. This is the minimum required data to achieve a successful result.
{
"author": "urn:li:person:___user_identifier___',
"commentary": "This is my first article posted from my Node.js app"
"visibility": "PUBLIC",
"distribution": {
"feedDistribution": "MAIN_FEED",
"targetEntities": [],
"thirdPartyDistributionChannels": []
},
"lifecycleState": "PUBLISHED",
}
In JavaScript, a complete, typical example using the fetch package is displayed below:
var fetch = require("node-fetch")
const response = await fetch("https://api.linkedin.com/rest/post", {
method: "POST",
header: {
'LinkedIn-Version': '202210',
'X-Restli-Protocol-Version': '2.0.0',
'Authorization': ' Bearer ___access_token___'
},
body: JSON.stringify({
"author": "urn:li:person:___user_identifier___',
"commentary": "This is my first article posted from my Node.js app"
"visibility": "PUBLIC",
"distribution": {
"feedDistribution": "MAIN_FEED",
"targetEntities": [],
"thirdPartyDistributionChannels": []
},
"lifecycleState": "PUBLISHED",
}))
```
Upon a successful request, you get a 201 (created) status and the x-restli-id
response header has the new postβs URN, urn:li:share:{ID}
. Here a screenshot of a successful API (from my IDE debugger):
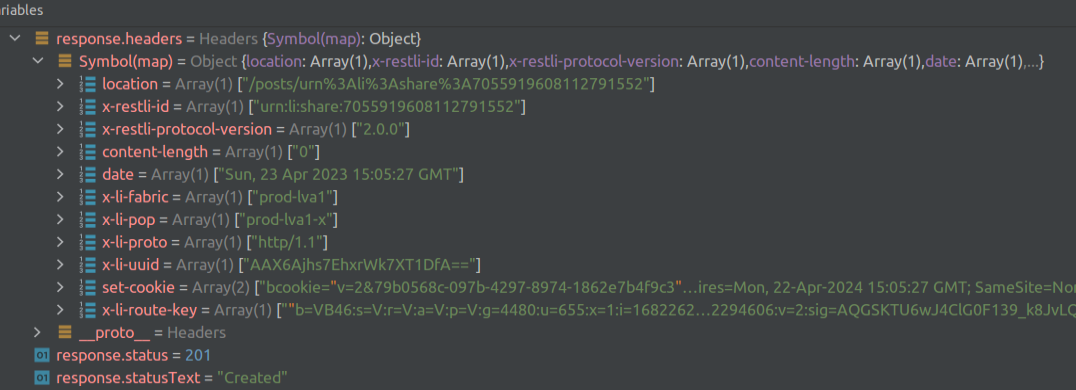
And here is the post in my LinkedIn account:
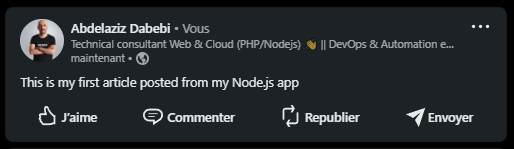
That's it. We've done it π
Conclusion
In this article, we went through an example of how to use the LinkedIn API access token and user identifier that we generated in the previous part of this series to automatically post on LinkedIn. I hope this was helpful. Don't hesitate to reach out to me if things don't work out for you. Happy posting!